Smart Contract Security
Understanding vulnerabilities and best practices for secure smart contracts.
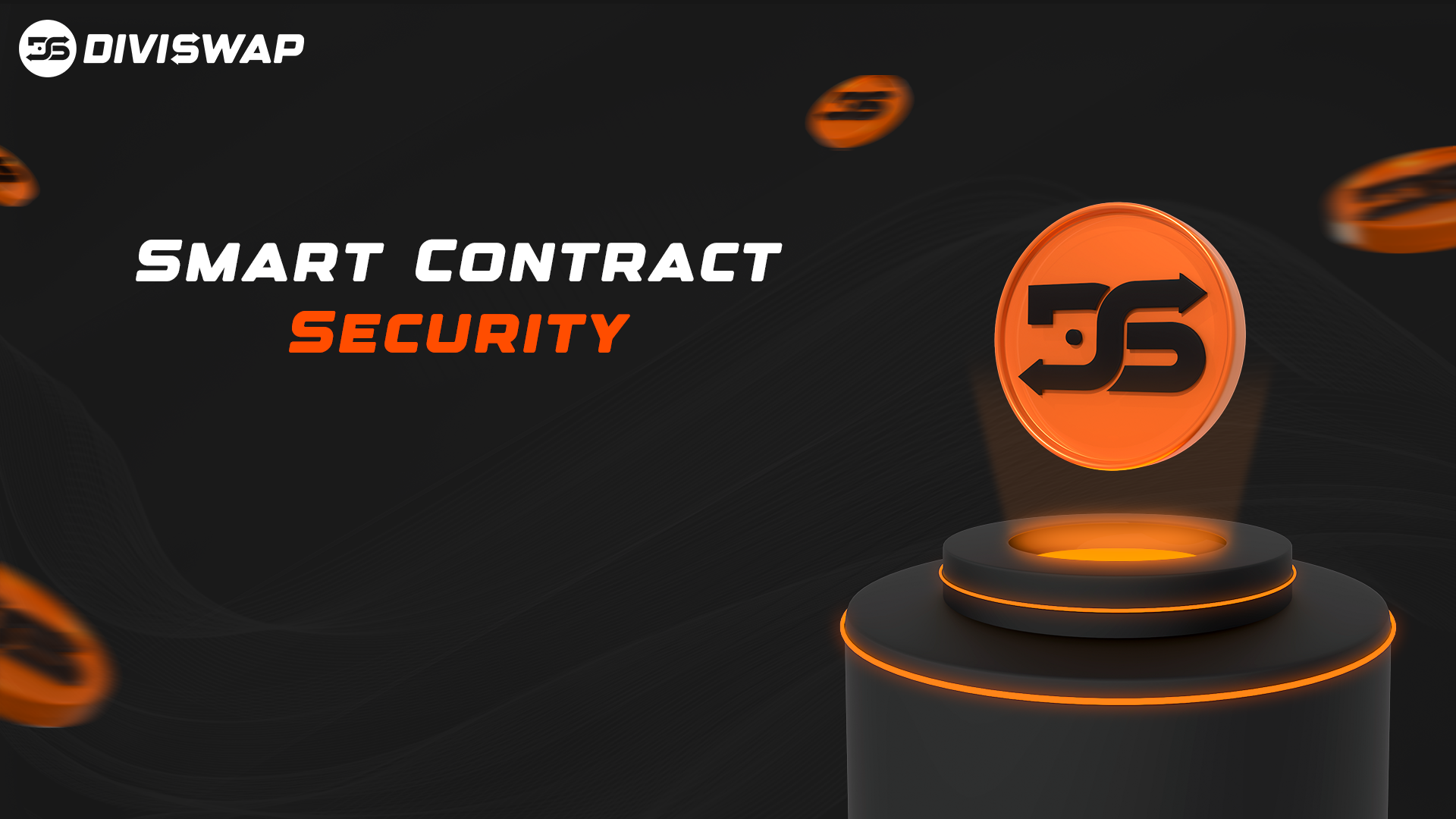
Common Smart Contract Vulnerabilities
Smart contract security is a critical aspect of blockchain development. As immutable code that controls digital assets, smart contracts must be developed with security as a top priority. This guide explores common vulnerabilities and best practices for securing smart contracts.
1. Reentrancy Attacks
Reentrancy occurs when external contract calls are allowed to make new calls to the calling contract before the first execution is complete. This can lead to functions being called repeatedly before the first call is complete, potentially draining funds.
// Vulnerable code
function withdraw(uint amount) public {
require(balances[msg.sender] >= amount);
(bool success, ) = msg.sender.call{value: amount}("");
require(success);
balances[msg.sender] -= amount;
}
Solution: Implement the checks-effects-interactions pattern, where state changes happen before external calls.
// Secure code
function withdraw(uint amount) public {
require(balances[msg.sender] >= amount);
balances[msg.sender] -= amount; // State change before external call
(bool success, ) = msg.sender.call{value: amount}("");
require(success);
}
2. Integer Overflow and Underflow
Before Solidity 0.8.0, arithmetic operations could overflow or underflow without reverting, leading to unexpected behavior.
Solution: Use Solidity 0.8.0+ which includes built-in overflow/underflow checks, or use SafeMath library for earlier versions.
3. Access Control Vulnerabilities
Improper access controls can allow unauthorized users to execute sensitive functions.
Solution: Implement proper modifiers and role-based access control.
// Access control example
modifier onlyOwner() {
require(msg.sender == owner, "Not authorized");
_;
}
function withdrawFunds() public onlyOwner {
// Function logic
}
Security Best Practices
1. Code Audits
Always have your smart contracts audited by reputable security firms before deployment. Multiple audits provide additional security.
2. Formal Verification
Formal verification mathematically proves that a contract behaves as expected under all possible scenarios.
3. Comprehensive Testing
Implement thorough unit tests, integration tests, and scenario-based tests to cover all possible execution paths.
4. Use Established Libraries
Leverage battle-tested libraries like OpenZeppelin for standard contract functionality rather than implementing from scratch.
5. Implement Emergency Stops
Include circuit breakers that can pause contract functionality in case vulnerabilities are discovered.
// Emergency stop pattern
bool public paused = false;
modifier whenNotPaused() {
require(!paused, "Contract is paused");
_;
}
function pause() public onlyOwner {
paused = true;
}
function unpause() public onlyOwner {
paused = false;
}
Security Tools for Smart Contract Development
- Slither: Static analysis framework to detect common vulnerabilities
- MythX: Security analysis platform for Ethereum smart contracts
- Echidna: Fuzzing tool for Ethereum smart contracts
- Manticore: Symbolic execution tool for smart contract security analysis
Conclusion
Smart contract security is an ongoing process that requires vigilance at every stage of development. By understanding common vulnerabilities and implementing security best practices, developers can significantly reduce the risk of exploits and protect user funds. Remember that security is not a one-time effort but a continuous commitment to maintaining and improving the safety of your smart contracts.
Ready to Learn More?
Continue your crypto education with these related articles: